Basic usage of tool use (function calling)
Overview
Tool use is a technique which allows developers to connect Cohere’s Command family models to external tools like search engines, APIs, functions, databases, etc.
This opens up a richer set of behaviors by leveraging tools to access external data sources, taking actions through APIs, interacting with a vector database, querying a search engine, etc., and is particularly valuable for enterprise developers, since a lot of enterprise data lives in external sources.
The Chat endpoint comes with built-in tool use capabilities such as function calling, multi-step reasoning, and citation generation.
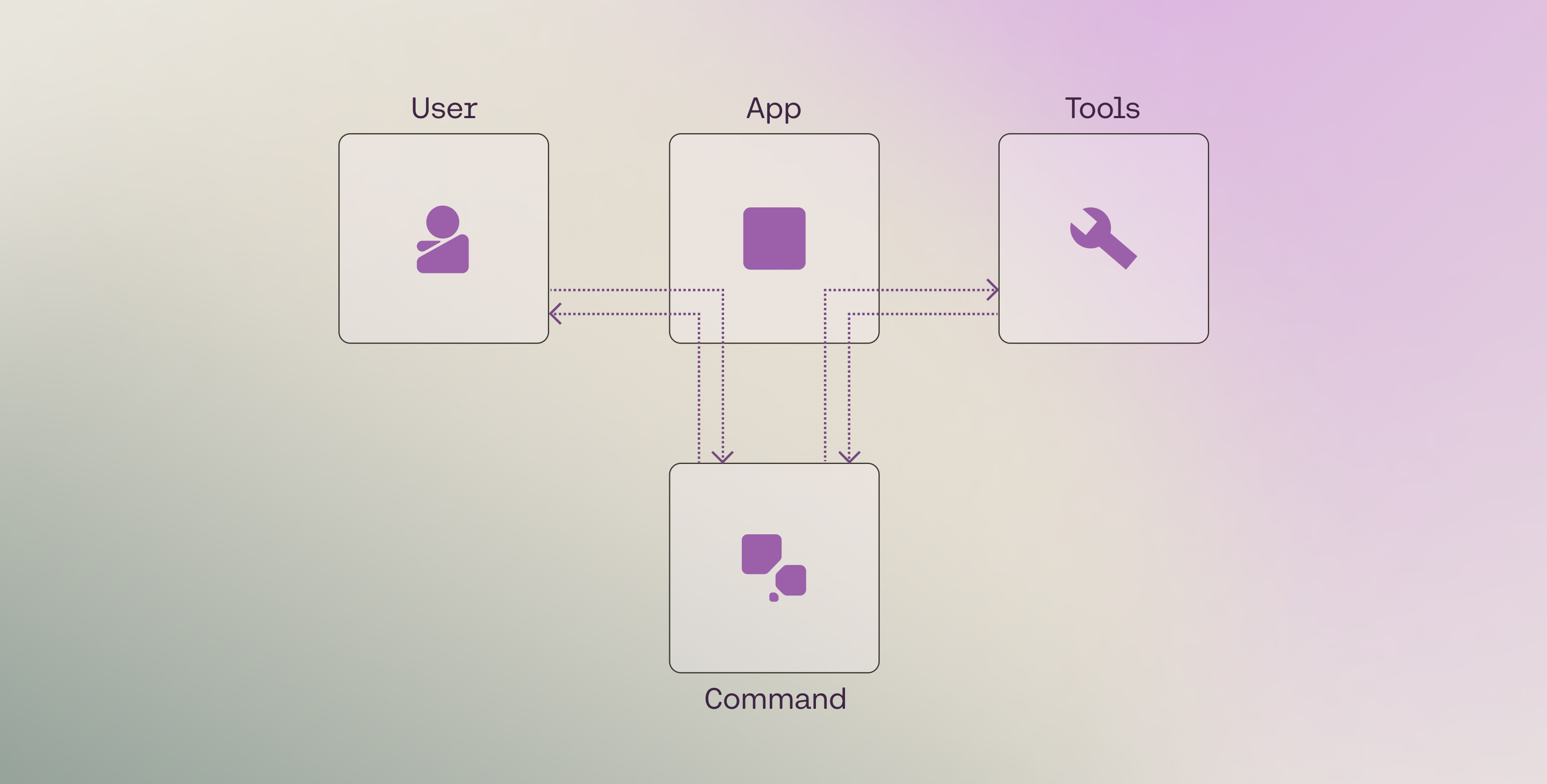
Setup
First, import the Cohere library and create a client.
Cohere platform
Private deployment
Tool definition
The pre-requisite, or Step 0, before we can run a tool use workflow, is to define the tools. We can break this further into two steps:
- Creating the tool
- Defining the tool schema
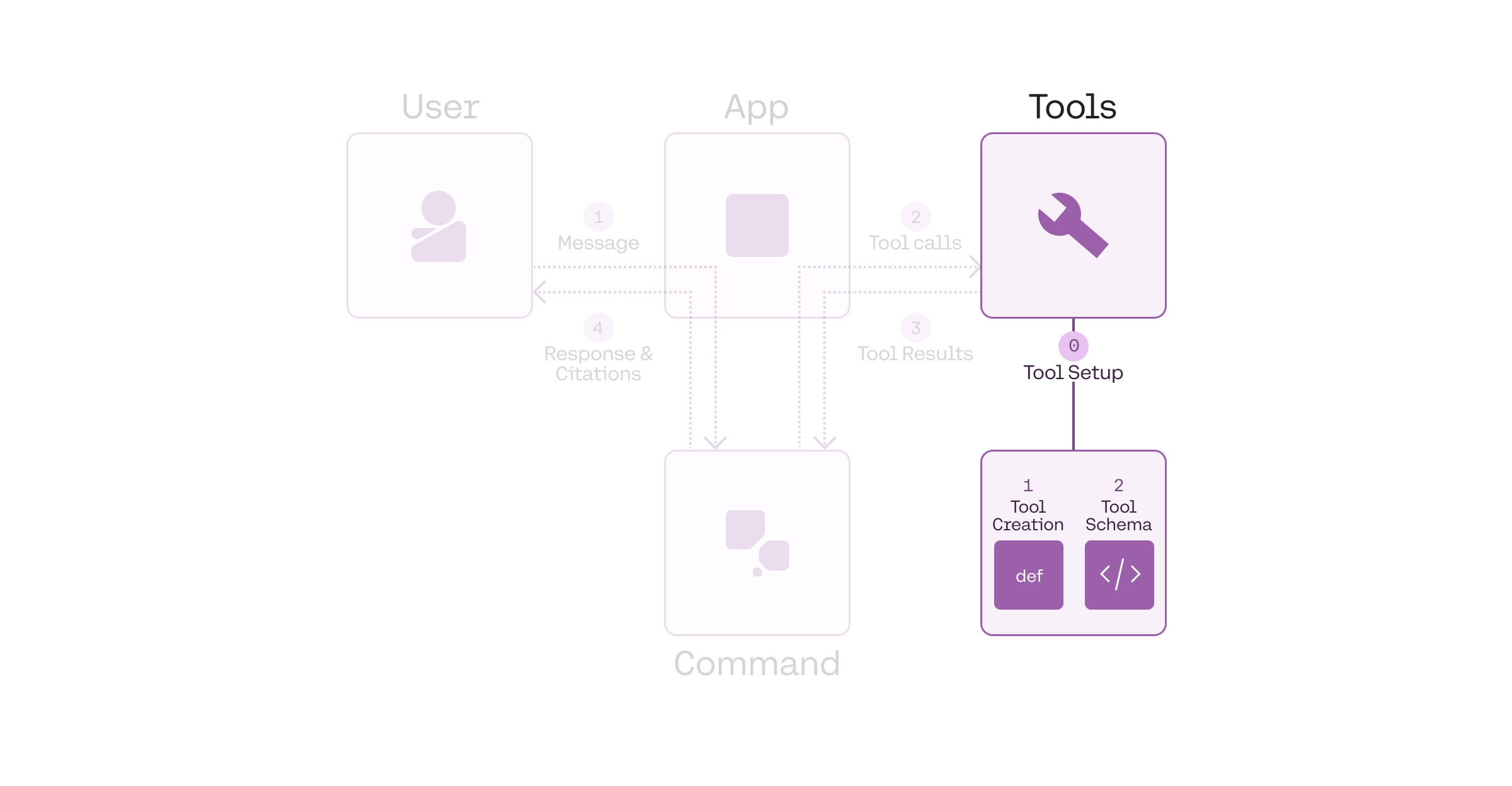
Creating the tool
A tool can be any function that you create or external services that return an object for a given input. Some examples: a web search engine, an email service, an SQL database, a vector database, a weather data service, a sports data service, or even another LLM.
In this example, we define a get_weather
function that returns the temperature for a given query, which is the location. You can implement any logic here, but to simplify the example, here we are hardcoding the return value to be the same for all queries.
The Chat endpoint accepts a string or a list of objects as the tool results. Thus, you should format the return value in this way. The following are some examples.
Defining the tool schema
We also need to define the tool schemas in a format that can be passed to the Chat endpoint. The schema follows the JSON Schema specification and must contain the following fields:
name
: the name of the tool.description
: a description of what the tool is and what it is used for.parameters
: a list of parameters that the tool accepts. For each parameter, we need to define the following fields:type
: the type of the parameter.properties
: the name of the parameter and the following fields:type
: the type of the parameter.description
: a description of what the parameter is and what it is used for.
required
: a list of required properties by name, which appear as keys in theproperties
object
This schema informs the LLM about what the tool does, and the LLM decides whether to use a particular tool based on the information that it contains.
Therefore, the more descriptive and clear the schema, the more likely the LLM will make the right tool call decisions.
In a typical development cycle, some fields such as name
, description
, and properties
will likely require a few rounds of iterations in order to get the best results (a similar approach to prompt engineering).
Tool use workflow
We can think of a tool use system as consisting of four components:
- The user
- The application
- The LLM
- The tools
At its most basic, these four components interact in a workflow through four steps:
- Step 1: Get user message: The LLM gets the user message (via the application).
- Step 2: Generate tool calls: The LLM decides which tools to call (if any) and generates the tool calls.
- Step 3: Get tool results: The application executes the tools, and the results are sent to the LLM.
- Step 4: Generate response and citations: The LLM generates the response and citations back to the user.
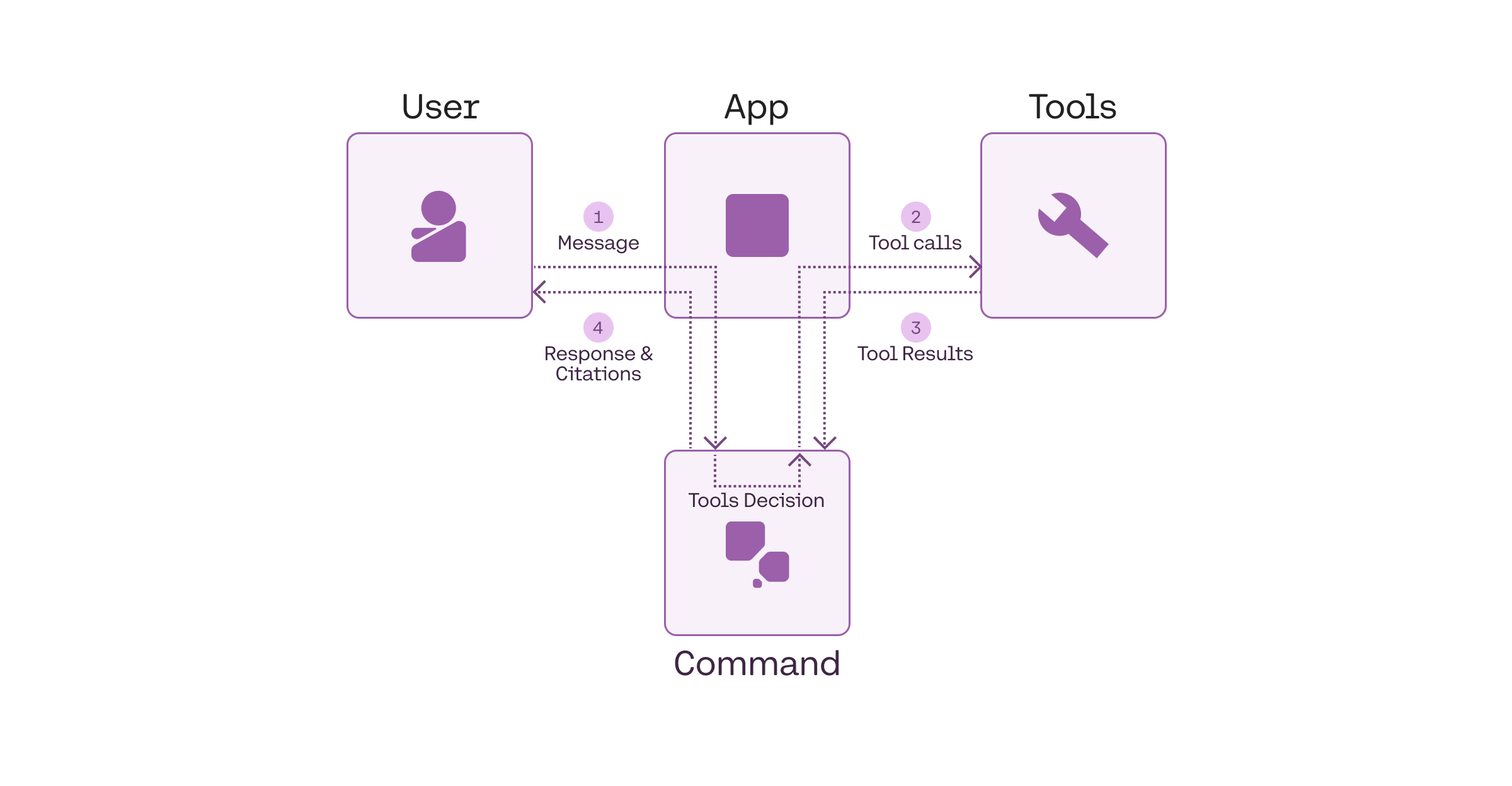
As an example, a weather search workflow might looks like the following:
- Step 1: Get user message: A user asks, “What’s the weather in Toronto?”
- Step 2: Generate tool calls: A tool call is made to an external weather service with something like
get_weather(“toronto”)
. - Step 3: Get tool results: The weather service returns the results, e.g. “20°C”.
- Step 4: Generate response and citations: The model provides the answer, “The weather in Toronto is 20 degrees Celcius”.
The following sections go through the implementation of these steps in detail.
Step 1: Get user message
In the first step, we get the user’s message and append it to the messages
list with the role
set to user
.
System message
Optional: If you want to define a system message, you can add it to the messages
list with the role
set to system
.
Step 2: Generate tool calls
Next, we call the Chat endpoint to generate the list of tool calls. This is done by passing the parameters model
, messages
, and tools
to the Chat endpoint.
The endpoint will send back a list of tool calls to be made if the model determines that tools are required. If it does, it will return two types of information:
tool_plan
: its reflection on the next steps it should take, given the user query.tool_calls
: a list of tool calls to be made (if any), together with auto-generated tool call IDs. Each generated tool call contains:id
: the tool call IDtype
: the type of the tool call (function
)function
: the function to be called, which contains the function’sname
andarguments
to be passed to the function.
We then append these to the messages
list with the role
set to assistant
.
Example response:
By default, when using the Python SDK, the endpoint passes the tool calls as objects of type ToolCallV2
and ToolCallV2Function
. With these, you get built-in type safety and validation that helps prevent common errors during development.
Alternatively, you can use plain dictionaries to structure the tool call message.
These two options are shown below.
Python objects
Plain dictionaries
Directly responding
The model can decide to not make any tool call, and instead, respond to a user message directly. This is described here.
Parallel tool calling
The model can determine that more than one tool call is required. This can be calling the same tool multiple times or different tools for any number of calls. This is described here.
Step 3: Get tool results
During this step, we perform the function calling. We call the necessary tools based on the tool call payloads given by the endpoint.
For each tool call, we append the messages
list with:
- the
tool_call_id
generated in the previous step. - the
content
of each tool result with the following fields:type
which isdocument
document
containingdata
: which stores the contents of the tool result.id
(optional): you can provide each document with a unique ID for use in citations, otherwise auto-generated
Step 4: Generate response and citations
By this time, the tool call has already been executed, and the result has been returned to the LLM.
In this step, we call the Chat endpoint to generate the response to the user, again by passing the parameters model
, messages
(which has now been updated with information fromthe tool calling and tool execution steps), and tools
.
The model generates a response to the user, grounded on the information provided by the tool.
We then append the response to the messages
list with the role
set to assistant
.
Example response:
It also generates fine-grained citations, which are included out-of-the-box with the Command family of models. Here, we see the model generating two citations, one for each specific span in its response, where it uses the tool result to answer the question.
Example response:
Multi-step tool use (agents)
Above, we assume the model performs tool calling only once (either single call or parallel calls), and then generates its response. This is not always the case: the model might decide to do a sequence of tool calls in order to answer the user request. This means that steps 2 and 3 will run multiple times in loop. It is called multi-step tool use and is described here.
State management
This section provides a more detailed look at how the state is managed via the messages
list as described in the tool use workflow above.
At each step of the workflow, the endpoint requires that we append specific types of information to the messages
list. This is to ensure that the model has the necessary context to generate its response at a given point.
In summary, each single turn of a conversation that involves tool calling consists of:
- A
user
message containing the user messagecontent
- An
assistant
message, containing the tool calling informationtool_plan
tool_calls
id
type
function
(consisting ofname
andarguments
)
- A
tool
message, containing the tool resultstool_call_id
content
containing a list of documents where each document contains the following fields:type
document
(consisting ofdata
and optionallyid
)
- A final
assistant
message, containing the model’s responsecontent
These correspond to the four steps described above. The list of messages
is shown below.
The sequence of messages
is represented in the diagram below.
Note that this sequence represents a basic usage pattern in tool use. The next page describes how this is adapted for other scenarios.