A Data Analyst Agent Built with Cohere and Langchain
Tool use is a method which allows developers to connect Cohere’s Command models to external tools like search engines, APIs, databases, and other software tools. Just like how Retrieval-Augmented Generation (RAG) allows a model to use an external data source to improve factual generation, tool use is a capability that allows retrieving data from multiple sources. But it goes beyond simply retrieving information and is able to use software tools to execute code, or even create entries in a CRM system.
In this notebook, we’ll see how we can use two tools to create a simple data analyst agent that is able to search the web and run code in a python interpreter. This agent uses Cohere’s Command R+ mode and Langchain.
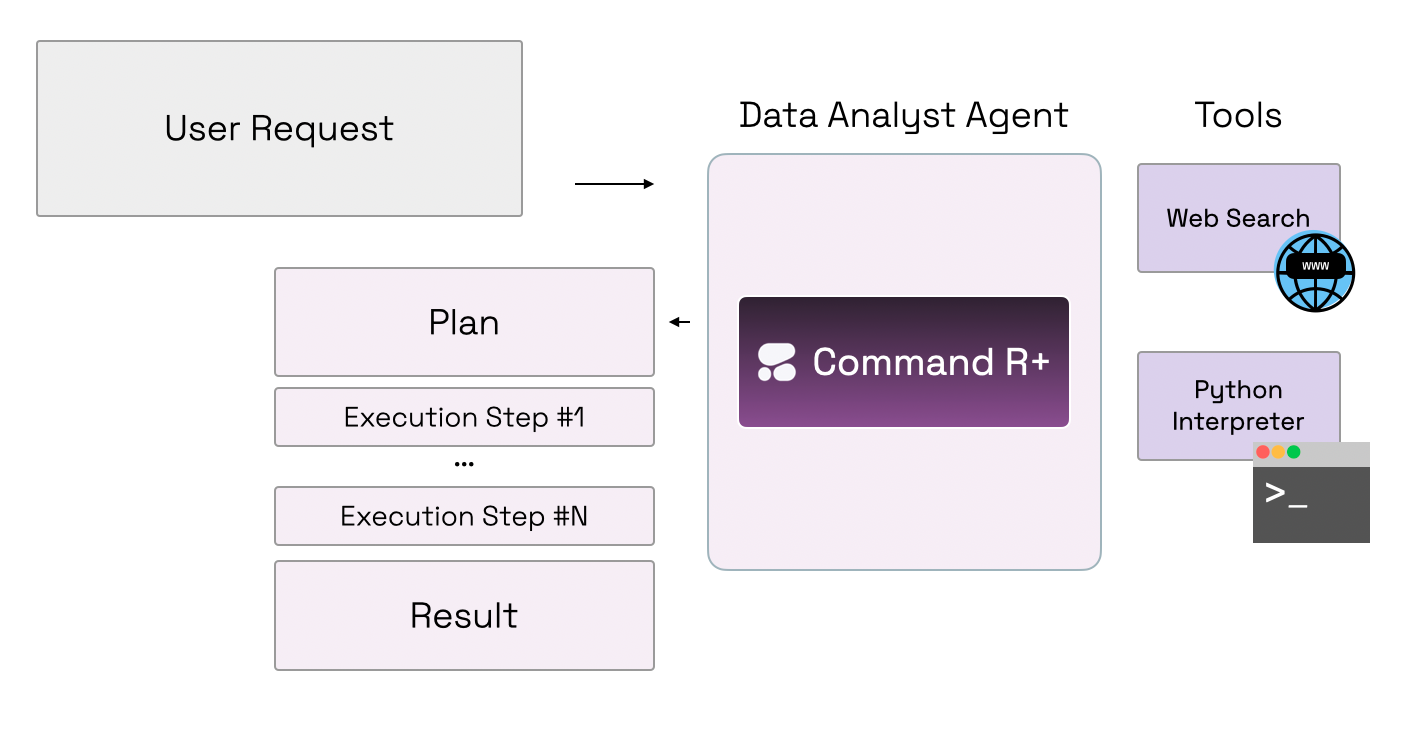
Let’s start by installing the required libraries
1 ! pip install --quiet langchain langchain_cohere langchain_experimental
Setup
We’ll need a Cohere API key here. Grab your key and paste it in the next slide if you have one, or register and create a new API key.
1 ### LLMs 2 import os 3 os.environ['COHERE_API_KEY'] = "<insert api="" cohere="" here="" key="">"
1 from langchain_cohere.chat_models import ChatCohere 2 chat = ChatCohere(model="command-a-03-2025", temperature=0.3)
Our simple data analyst will be equipped with a web search tool, and a python interpreter (which we can use to run plotting code, for example).
Web search
Let’s first equip our agent with web search! We can use the Tavily API for this. Head on to tavily.com and grab an API key to use here.
1 from langchain_community.tools.tavily_search import TavilySearchResults 2 3 os.environ['TAVILY_API_KEY'] = "<insert api="" here="" key="" tavily="">" 4 5 internet_search = TavilySearchResults() 6 internet_search.name = "internet_search" 7 internet_search.description = "Returns a list of relevant document snippets for a textual query retrieved from the internet." 8 9 10 from langchain_core.pydantic_v1 import BaseModel, Field 11 class TavilySearchInput(BaseModel): 12 query: str = Field(description="Query to search the internet with") 13 internet_search.args_schema = TavilySearchInput
Python interpreter tool
Let’s equip our agent with a python interpreter!
1 from langchain.agents import Tool 2 from langchain_experimental.utilities import PythonREPL 3 4 python_repl = PythonREPL() 5 repl_tool = Tool( 6 name="python_repl", 7 description="Executes python code and returns the result. The code runs in a static sandbox without interactive mode, so print output or save output to a file.", 8 func=python_repl.run, 9 ) 10 repl_tool.name = "python_interpreter" 11 12 class ToolInput(BaseModel): 13 code: str = Field(description="Python code to execute.") 14 repl_tool.args_schema = ToolInput
1 from langchain.agents import AgentExecutor 2 from langchain_cohere.react_multi_hop.agent import create_cohere_react_agent 3 from langchain_core.prompts import ChatPromptTemplate
1 prompt = ChatPromptTemplate.from_template("{input}") 2 3 4 agent = create_cohere_react_agent( 5 llm=chat, 6 tools=[internet_search, repl_tool], 7 prompt=prompt, 8 )
1 agent_executor = AgentExecutor(agent=agent, tools=[internet_search, repl_tool], verbose=True)
1 agent_executor.invoke({ 2 "input": "Create a plot of the number of full time employees at the 3 tech companies with the highest market cap in the United States in 2024.", 3 })
[1m> Entering new AgentExecutor chain...[0m [32;1m[1;3m First, I will search for the three tech companies with the highest market cap in the US in 2024. Then, I will search for the number of full-time employees at each of these companies, and plot the data using Python. {'tool_name': 'internet_search', 'parameters': {'query': 'top 3 tech companies highest market cap US 2024'}} [0m[36;1m[1;3m[{'url': 'https://www.fool.com/research/largest-companies-by-market-cap/', 'content': "It's the most valuable automaker in the world and has the world's best-selling car in the Model Y.\nTesla is most famous for its vehicles, and it's second only to China's BYD Company (OTC:BYDDY) among the largest EV companies in terms of manufacturing. While it's most famous for Windows, Microsoft also has a diverse selection of products and services that has helped to build on its success, including:\nMicrosoft has been the world's largest company before, and it briefly surpassed Apple for the biggest market cap in 2021. Walmart\nWalmart (NYSE:WMT) may not have the largest market cap, but it is No. 1 in terms of revenue, and it’s the largest retailer in the world. Microsoft\nConsidering the popularity of the Windows operating system, it’s no surprise that Microsoft (NASDAQ:MSFT) has consistently ranked as one of the largest companies in the world. Although the top spot has changed hands on multiple occasions, Apple has spent the most time there and is currently the most valuable company in the world.\n"}, {'url': 'https://www.financecharts.com/screener/biggest-country-us', 'content': 'Biggest Companies in the US by Market Cap for Apr 2024. The most valuable company in the US is Microsoft (MSFT) with a market cap of $3.159T, followed by Apple (AAPL) and NVIDIA (NVDA). Last updated Apr 05, 2024.'}, {'url': 'https://www.statista.com/statistics/1350976/leading-tech-companies-worldwide-by-market-cap/', 'content': 'Digital & Trend reports\nOverview and forecasts on trending topics\nIndustry & Market reports\nIndustry and market insights and forecasts\nCompanies & Products reports\nKey figures and rankings about companies and products\nConsumer & Brand reports\nConsumer and brand insights and preferences in various industries\nPolitics & Society reports\nDetailed information about political and social topics\nCountry & Region reports\nAll key figures about countries and regions\nMarket forecast and expert KPIs for 1000+ markets in 190+ countries & territories\nInsights on consumer attitudes and behavior worldwide\nBusiness information on 100m+ public and private companies\nExplore Company Insights\nDetailed information for 39,000+ online stores and marketplaces\nDirectly accessible data for 170 industries from 150+ countries\nand over 1\xa0Mio. facts.\n Other statistics on the topicAI chips\nHardware\nLeading semiconductor companies worldwide 2023, by market cap\nHardware\nSemiconductor market revenue growth worldwide 1988-2024\nHardware\nNvidia revenue worldwide 2015-2023, by segment\nHardware\nSemiconductor market size worldwide 2020-2030, by application\nYou only have access to basic statistics.\n Other statistics that may interest you\nOther statistics that may interest you Statistics on\nAbout the industry\nAbout the region\nOther regions\nRelated statistics\nFurther related statistics\nFurther Content: You might find this interesting as well\nStatistics\nTopics Transforming data into design:\nStatista Content & Design\nStrategy and business building for the data-driven economy:\nLeading tech companies worldwide 2023, by market cap\nApple\nSamsung\nLeading tech companies worldwide 2023, by market capitalization\n(in billion U.S. dollars)\n Additional Information\nShow sources information\nShow publisher information\nUse Ask Statista Research Service\nAugust 2023\nWorldwide\n2023\n'}, {'url': 'https://www.forbes.com/advisor/investing/best-tech-stocks/', 'content': 'eToro\nThe Best Tech Stocks of November 2023\nApple Inc. (AAPL)\n$2.8 trillion\n0.5%\n27.1%\n$2.8 trillion\n0.5%\n27.1%\nApple\xa0 was founded in Los Altos, Calif., by Steve Jobs and Steve Wozniak in 1976. ASML Holding NV (ASML)\n$248 billion\n1.0%\n22.8%\n$248 billion\n1.0%\n22.8%\nASML is a Netherlands-based company that designs and manufactures the machinery used by companies that make microchips. Taiwan Semiconductor Manufacturing Company (TSM)\n$443 billion\n2.0%\n20.6%\n$443 billion\n2.0%\n20.6%\nTaiwan Semiconductor Manufacturing Company could be the biggest tech company you’ve never heard of. NVIDIA Corp (NVDA)\n$1.1 trillion\n0.0%\n62.8%\n$1.1 trillion\n0.0%\n62.8%\nNVIDIA was founded in 1993 to produce graphic cards for the burgeoning personal computer market. Microsoft Corporation (MSFT)\n$2.6 trillion\n0.8%\n27.3%\n$2.6 trillion\n0.8%\n27.3%\nMicrosoft\xa0 was founded in 1975 by Bill Gates and Paul Allen in Albuquerque, N.M.'}, {'url': 'https://disfold.com/united-states/sector/technology/companies/', 'content': 'Unlock Financial AI:\nStart your Free Trial of\nDisfold AI, Now!\nTop Technology Companies from the United States as of Jan. 01, 2024\n(Dec. 1, 2023)\nTop Technology Companies from the United States as of Jan. 01, 2024\n1.\nApple\nMarket Cap (USD):\n$2.866 T\nStock:\nAAPL\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nConsumer Electronics\n2.\nMicrosoft\nMarket Cap (USD):\n$2.755 T\nStock:\nMSFT\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n3.\nNvidia\nMarket Cap (USD):\n$1.186 T\nStock:\nNVDA\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n4.\nBroadcom\nMarket Cap (USD):\n$495.95 B\nStock:\nAVGO\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n5.\nOracle\nMarket Cap (USD):\n$282.01 B\nStock:\nORCL\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n6.\n Marvell Technology, Inc.\nMarket Cap (USD):\n$49.61 B\nStock:\n9MW\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n35.\nAutodesk, Inc.\nMarket Cap (USD):\n$48.97 B\nStock:\nADSK\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Application\n36.\nMicrochip Technology Incorporated\nMarket Cap (USD):\n$45.77 B\nStock:\nMCHP\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n37.\nFortinet, Inc.\nMarket Cap (USD):\n$44.84 B\nStock:\n Analog Devices\nMarket Cap (USD):\n$93.79 B\nStock:\nADI\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n20.\nMicron Technology, Inc.\nMarket Cap (USD):\n$91.30 B\nStock:\nMU\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n21.\nPalo Alto Networks\nMarket Cap (USD):\n$90.41 B\nStock:\nPANW\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n22.\n Fiserv\nMarket Cap (USD):\n$79.73 B\nStock:\nFI\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nInformation Technology Services\n23.\nKLA\nMarket Cap (USD):\n$75.13 B\nStock:\nKLAC\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductor Equipment & Materials\n24.\nSynopsys\nMarket Cap (USD):\n$74.64 B\nStock:\nSNPS\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n25.\n Salesforce\nMarket Cap (USD):\n$243.78 B\nStock:\nCRM\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Application\n8.\nAMD\nMarket Cap (USD):\n$219.72 B\nStock:\nAMD\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n9.\nCisco\nMarket Cap (USD):\n$205.21 B\nStock:\nCSCO\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nCommunication Equipment\n10.\n'}][0m[32;1m[1;3m I have found that the three tech companies with the highest market cap in the US in 2024 are Microsoft, Apple and NVIDIA. Now, I will search for the number of full-time employees at each of these companies and plot the data. {'tool_name': 'internet_search', 'parameters': {'query': 'Microsoft full time employees 2024'}} [0m[36;1m[1;3m[{'url': 'https://www.statista.com/statistics/273475/number-of-employees-at-the-microsoft-corporation-since-2005/', 'content': 'Digital & Trend reports\nOverview and forecasts on trending topics\nIndustry & Market reports\nIndustry and market insights and forecasts\nCompanies & Products reports\nKey figures and rankings about companies and products\nConsumer & Brand reports\nConsumer and brand insights and preferences in various industries\nPolitics & Society reports\nDetailed information about political and social topics\nCountry & Region reports\nAll key figures about countries and regions\nMarket forecast and expert KPIs for 1000+ markets in 190+ countries & territories\nInsights on consumer attitudes and behavior worldwide\nBusiness information on 100m+ public and private companies\nExplore Company Insights\nDetailed information for 39,000+ online stores and marketplaces\nDirectly accessible data for 170 industries from 150+ countries\nand over 1\xa0Mio. facts.\n Other statistics on the topicMicrosoft\nSoftware\nMicrosoft\'s expenditure on research and development 2002-2023\nSoftware\nOffice productivity software market share worldwide 2022\nIT Services\nCloud infrastructure services market share quarterly worldwide 2017-2022, by vendor\nSoftware\nMicrosoft\'s revenue 2008-2024, by fiscal quarter\nTo download this statistic in XLS format you need a Statista Account\nTo download this statistic in PNG format you need a Statista Account\nTo download this statistic in PDF format you need a Statista Account\nTo download this statistic in PPT format you need a Statista Account\nAs a Premium user you get access to the detailed source references and background information about this statistic.\n Statistics on\n"\nMicrosoft\n"\nOther statistics that may interest you Microsoft\nOverview\nCompany financials\nSegments\nCompetitors: Software\nCompetitors: Cloud\nCompetitors: Devices\nFurther related statistics\nFurther Content: You might find this interesting as well\nStatistics\nTopics Number of Microsoft employees 2005-2023\nHow many employees does Microsoft have?\nNumber of employees at the Microsoft Corporation from 2005 to 2023\n(in 1,000s)\nAdditional Information\nShow sources information\nShow publisher information\nUse Ask Statista Research Service\nJuly 2023\nWorldwide\n2005 to 2023\nMicrosoft\'s fiscal year ends on June 30.\n Transforming data into design:\nStatista Content & Design\nStrategy and business building for the data-driven economy:\nIndustry-specific and extensively researched technical data (partially from exclusive partnerships).'}, {'url': 'https://stockanalysis.com/stocks/msft/employees/', 'content': "MSFT News. 1 day ago - Microsoft Pushes LinkedIn Further Into the Connected Economy - PYMNTS 1 day ago - Softchoice to Advance Generative AI and Security Solutions Through New Agreement With Microsoft - Business Wire 1 day ago - AMD, Samsung, Check Point, Microsoft, and Other Tech Stocks in Focus Today - Barrons 1 day ago - Don't chase the value rebound, tech still has a few surprises up its ..."}, {'url': 'https://www.macrotrends.net/stocks/charts/MSFT/microsoft/number-of-employees', 'content': 'Interactive chart of Microsoft (MSFT) annual worldwide employee count from 2010 to 2023. Microsoft total number of employees in 2023 was 221,000, a 0% decline from 2022. Microsoft total number of employees in 2022 was 221,000, a 22.1% increase from 2021. Microsoft total number of employees in 2021 was 181,000, a 11.04% increase from 2020.'}, {'url': 'https://www.microsoft.com/investor/reports/ar23/index.html', 'content': 'As of June 30, 2023, we employed approximately 221,000 people on a full-time basis, 120,000 in the U.S. and 101,000 internationally. Of the total employed people, 89,000 were in operations, including manufacturing, distribution, product support, and consulting services; 72,000 were in product research and development; 45,000 were in sales and ...'}, {'url': 'https://www.microsoft.com/en-us/investor/earnings/fy-2024-q2/press-release-webcast', 'content': 'Microsoft Cloud Strength Drives Second Quarter Results. REDMOND, Wash. — January 30, 2024 — Microsoft Corp. today announced the following results for the quarter ended December 31, 2023, as compared to the corresponding period of last fiscal year: · Revenue was $62.0 billion and increased 18% (up 16% in constant currency) · Operating income was $27.0 billion and increased 33%, and ...'}][0m[32;1m[1;3m {'tool_name': 'internet_search', 'parameters': {'query': 'Apple full time employees 2024'}} [0m[36;1m[1;3m[{'url': 'https://www.macrotrends.net/stocks/charts/AAPL/apple/number-of-employees', 'content': 'Employee Count. Interactive chart of Apple (AAPL) annual worldwide employee count from 2010 to 2023. Apple total number of employees in 2023 was 161,000, a 1.83% decline from 2022. Apple total number of employees in 2022 was 164,000, a 6.49% increase from 2021. Apple total number of employees in 2021 was 154,000, a 4.76% increase from 2020.'}, {'url': 'https://www.businessinsider.com/apple-layoffs-tim-cook-getting-serious-challenges-car-china-iphone-2024-4?op=1', 'content': "2024-04-05T12:27:33Z An curved arrow pointing right. Share. The ... That's especially so when considering that Apple had about 161,000 full-time employees at the end of its last fiscal year."}, {'url': 'https://www.statista.com/statistics/273439/number-of-employees-of-apple-since-2005/', 'content': 'Digital & Trend reports\nOverview and forecasts on trending topics\nIndustry & Market reports\nIndustry and market insights and forecasts\nCompanies & Products reports\nKey figures and rankings about companies and products\nConsumer & Brand reports\nConsumer and brand insights and preferences in various industries\nPolitics & Society reports\nDetailed information about political and social topics\nCountry & Region reports\nAll key figures about countries and regions\nMarket forecast and expert KPIs for 1000+ markets in 190+ countries & territories\nInsights on consumer attitudes and behavior worldwide\nBusiness information on 100m+ public and private companies\nExplore Company Insights\nDetailed information for 39,000+ online stores and marketplaces\nDirectly accessible data for 170 industries from 150+ countries\nand over 1\xa0Mio. facts.\n Other statistics on the topicApple\nConsumer Electronics\nApple\'s revenue worldwide 2004-2023\nConsumer Electronics\nApple\'s revenue broken down by geographical region 2012-2023, by quarter\nTelecommunications\nQuarterly market share of smartphone vendors in the U.S. 2016-2023\nConsumer Electronics\nApple: expenditure on research and development 2007-2023\nTo download this statistic in XLS format you need a Statista Account\nTo download this statistic in PNG format you need a Statista Account\nTo download this statistic in PDF format you need a Statista Account\nTo download this statistic in PPT format you need a Statista Account\nAs a Premium user you get access to the detailed source references and background information about this statistic.\n Statistics on\n"\nApple in the U.S.\n"\nOther statistics that may interest you Apple in the U.S.\nOverview: Apple\nOverview: Apple in the U.S.\nSegment: iPhone\nSegment: iPad\nSegment: Mac\nSegment: Wearables & Smart home\nFurther related statistics\nFurther Content: You might find this interesting as well\nStatistics\nTopics Number of employees of Apple 2005-2023\nApple Corporation – additional information\nApple products\nApple\'s number of employees in the fiscal years 2005 to 2023\n(in 1,000s)\nAdditional Information\nShow sources information\nShow publisher information\nUse Ask Statista Research Service\nNovember 2023\nWorldwide\n2005 to 2023\n Transforming data into design:\nStatista Content & Design\nStrategy and business building for the data-driven economy:\nIndustry-specific and extensively researched technical data (partially from exclusive partnerships).'}, {'url': 'https://jobs.apple.com/en-us/details/200542480/fy-24-full-time-opportunity-for-fresh-graduates-general', 'content': "Shop and Learn\nServices\nAccount\nApple Store\nFor Business\nFor Education\nFor Healthcare\nFor Government\nApple Values\nAbout Apple Summary\nKey Qualifications\nDescription\nEducation & Experience\nAdditional Requirements\nAdd a favorite\nDon’t have an Apple ID?\nApple Footer\nApple is an equal opportunity employer that is committed to inclusion and diversity. If you’re applying for a position in San Francisco,review the\nSan Francisco Fair Chance Ordinance guidelines (opens in a new window)\napplicable in your area.\n FY'24 Full Time Opportunity for Fresh Graduates - General\nAdd a favorite\nDon’t have an Apple ID?\n Learn more about your EEO rights as an applicant (Opens in a new window) .\n"}, {'url': 'https://jobs.apple.com/en-us/details/200544662/wiml-2024-full-time-opportunities-at-apple', 'content': 'Shop and Learn\nServices\nAccount\nApple Store\nFor Business\nFor Education\nFor Healthcare\nFor Government\nApple Values\nAbout Apple Summary\nKey Qualifications\nDescription\nEducation & Experience\nAdditional Requirements\nAdd a favorite\nDon’t have an Apple ID?\nApple Footer\nApple is an equal opportunity employer that is committed to inclusion and diversity. If you’re applying for a position in San Francisco,review the\nSan Francisco Fair Chance Ordinance guidelines (opens in a new window)\napplicable in your area.\n WiML 2024 - Full Time Opportunities at Apple\nAdd a favorite\nDon’t have an Apple ID?\n Learn more about your EEO rights as an applicant (Opens in a new window) .\n'}][0m[32;1m[1;3m {'tool_name': 'internet_search', 'parameters': {'query': 'NVIDIA full time employees 2024'}} [0m[36;1m[1;3m[{'url': 'https://www.macrotrends.net/stocks/charts/NVDA/nvidia/number-of-employees', 'content': 'Employee Count. Interactive chart of NVIDIA (NVDA) annual worldwide employee count from 2010 to 2024. NVIDIA total number of employees in 2024 was 29,600, a 12.99% increase from 2023. NVIDIA total number of employees in 2023 was 26,196, a 16.57% increase from 2022. NVIDIA total number of employees in 2022 was 22,473, a 18.43% increase from 2021.'}, {'url': 'https://www.statista.com/statistics/1369575/nvidia-number-of-employees-by-region/', 'content': 'Digital & Trend reports\nOverview and forecasts on trending topics\nIndustry & Market reports\nIndustry and market insights and forecasts\nCompanies & Products reports\nKey figures and rankings about companies and products\nConsumer & Brand reports\nConsumer and brand insights and preferences in various industries\nPolitics & Society reports\nDetailed information about political and social topics\nCountry & Region reports\nAll key figures about countries and regions\nMarket forecast and expert KPIs for 1000+ markets in 190+ countries & territories\nInsights on consumer attitudes and behavior worldwide\nBusiness information on 100m+ public and private companies\nExplore Company Insights\nDetailed information for 39,000+ online stores and marketplaces\nDirectly accessible data for 170 industries from 150+ countries\nand over 1\xa0Mio. facts.\n Other statistics on the topicNvidia\nHardware\nSemiconductor market revenue worldwide 1987-2024\nHardware\nLeading semiconductor companies worldwide 2024, by market cap\nHardware\nSemiconductor companies market revenue share worldwide 2008-2023\nHardware\nSemiconductor market revenue growth worldwide 1988-2024\nTo download this statistic in XLS format you need a Statista Account\nTo download this statistic in PNG format you need a Statista Account\nTo download this statistic in PDF format you need a Statista Account\nTo download this statistic in PPT format you need a Statista Account\nAs a Premium user you get access to the detailed source references and background information about this statistic.\n Nvidia number of employees 2023, by region\nNvidia number of employees in 2023, by region\nAdditional Information\nShow sources information\nShow publisher information\nUse Ask Statista Research Service\nJuly 2023\nWorldwide\n2023\nIncludes full-time and part-time employees.\n Statistics on\n"\nNvidia\n"\nOther statistics that may interest you Nvidia\nOverview\nFinancials\nSegments\nESG\nCompetitors\nFurther Content: You might find this interesting as well\nTopics Transforming data into design:\nStatista Content & Design\nStrategy and business building for the data-driven economy:\nIndustry-specific and extensively researched technical data (partially from exclusive partnerships).'}, {'url': 'https://finance.yahoo.com/news/nvidia-announces-financial-results-fourth-212000202.html', 'content': "Proceeds related to employee stock plans\n-\n5\n403\n355\nPayments related to repurchases of common stock\n(2,660\n)\n(1,212\n)\n(9,533\n)\n(10,039\n)\nPayments related to tax on restricted stock units\n(841\n)\n(344\n)\n(2,783\n)\n(1,475\n)\nRepayment of debt\n-\n-\n(1,250\n)\n-\nDividends paid\n(99\n)\n(98\n)\n(395\n)\n(398\n)\nPrincipal payments on property and equipment and intangible assets\n(29\n)\n(4\n)\n(74\n)\n(58\n)\nOther\n-\n(3\n)\n(1\n)\n(2\n)\nNet cash used in financing activities\n(3,629\n)\n(1,656\n)\n(13,633\n)\n(11,617\n)\nChange in cash and cash equivalents\n1,761\n589\n3,891\n1,399\nCash and cash equivalents at beginning of period\n5,519\n2,800\n3,389\n1,990\nCash and cash equivalents at end of period\n$\n7,280\n$\n3,389\n$\n7,280\n$\n3,389\nSupplemental disclosures of cash flow information:\nCash paid for income taxes, net\n$\n1,874\n$\n32\n$\n6,549\n$\n1,404\nCash paid for interest\n$\n26\n$\n28\n$\n252\n$\n254\nNVIDIA CORPORATION\nRECONCILIATION OF GAAP TO NON-GAAP FINANCIAL MEASURES\n(In millions, except per share data)\n(Unaudited)\nThree Months Ended\nTwelve Months Ended\nJanuary 28,\nOctober 29,\nJanuary 29,\nJanuary 28,\nJanuary 29,\n2024\n2023\n2023\n2024\n2023\nGAAP gross profit\n$\n16,791\n$\n13,400\n$\n3,833\n$\n44,301\n$\n15,356\nGAAP gross margin\n76.0\n%\n74.0\n%\n63.3\n%\n72.7\n%\n56.9\n%\nAcquisition-related and other costs (A)\n119\n119\n120\n477\n455\nStock-based compensation expense (B)\n45\n38\n30\n141\n138\nIP-related costs\n4\n26\n16\n40\n16\nNon-GAAP gross profit\n$\n16,959\n$\n13,583\n$\n3,999\n$\n44,959\n$\n15,965\nNon-GAAP gross margin\n76.7\n%\n75.0\n%\n66.1\n%\n73.8\n%\n59.2\n%\nGAAP operating expenses\n$\n3,176\n$\n2,983\n$\n2,576\n$\n11,329\n$\n11,132\nStock-based compensation expense (B)\n(948\n)\n(941\n)\n(709\n)\n(3,408\n)\n(2,572\n)\nAcquisition-related and other costs (A)\n(18\n)\n(16\n)\n(54\n)\n(106\n)\n(219\n)\nAcquisition termination cost\n-\n-\n-\n-\n(1,353\n)\nOther (C)\n-\n-\n(38\n)\n10\n(63\n)\nNon-GAAP operating expenses\n$\n2,210\n$\n2,026\n$\n1,775\n$\n7,825\n$\n6,925\nGAAP operating income\n$\n13,615\n$\n10,417\n$\n1,257\n$\n32,972\n$\n4,224\nTotal impact of non-GAAP adjustments to operating income\n1,134\n1,140\n967\n4,162\n4,816\nNon-GAAP operating income\n$\n14,749\n$\n11,557\n$\n2,224\n$\n37,134\n$\n9,040\nGAAP other income (expense), net\n$\n491\n$\n105\n$\n32\n$\n846\n$\n(43\n)\n(Gains) losses from non-affiliated investments\n(260\n)\n69\n10\n(238\n)\n45\nInterest expense related to amortization of debt discount\n1\n1\n1\n4\n5\nNon-GAAP other income (expense), net\n$\n232\n$\n175\n$\n43\n$\n612\n$\n7\nGAAP net income\n$\n12,285\n$\n9,243\n$\n1,414\n$\n29,760\n$\n4,368\nTotal pre-tax impact of non-GAAP adjustments\n875\n1,210\n978\n3,928\n4,865\nIncome tax impact of non-GAAP adjustments (D)\n(321\n)\n(433\n)\n(218\n)\n(1,376\n)\n(867\n)\n Other, net\n260\n(18\n)\n237\n(48\n)\nOther income (expense), net\n491\n32\n846\n(43\n)\nIncome before income tax\n14,106\n1,289\n33,818\n4,181\nIncome tax expense (benefit)\n1,821\n(125\n)\n4,058\n(187\n)\nNet income\n$\n12,285\n$\n1,414\n$\n29,760\n$\n4,368\nNet income per share:\nBasic\n$\n4.98\n$\n0.57\n$\n12.05\n$\n1.76\nDiluted\n$\n4.93\n$\n0.57\n$\n11.93\n$\n1.74\nWeighted average shares used in per share computation:\nBasic\n2,466\n2,464\n2,469\n2,487\nDiluted\n2,490\n2,477\n2,494\n2,507\nNVIDIA CORPORATION\nCONDENSED CONSOLIDATED BALANCE SHEETS\n(In millions)\n(Unaudited)\nJanuary 28,\nJanuary 29,\n2024\n2023\nASSETS\nCurrent assets:\nCash, cash equivalents and marketable securities\n$\n25,984\n$\n13,296\nAccounts receivable, net\n9,999\n3,827\nInventories\n5,282\n5,159\nPrepaid expenses and other current assets\n3,080\n791\nTotal current assets\n44,345\n23,073\nProperty and equipment, net\n3,914\n3,807\nOperating lease assets\n1,346\n1,038\nGoodwill\n4,430\n4,372\nIntangible assets, net\n1,112\n1,676\nDeferred income tax assets\n6,081\n3,396\nOther assets\n4,500\n3,820\nTotal assets\n$\n65,728\n$\n41,182\nLIABILITIES AND SHAREHOLDERS' EQUITY\nCurrent liabilities:\nAccounts payable\n$\n2,699\n$\n1,193\nAccrued and other current liabilities\n6,682\n4,120\nShort-term debt\n1,250\n1,250\nTotal current liabilities\n10,631\n6,563\nLong-term debt\n8,459\n9,703\nLong-term operating lease liabilities\n1,119\n902\nOther long-term liabilities\n2,541\n1,913\nTotal liabilities\n22,750\n19,081\nShareholders' equity\n42,978\n22,101\nTotal liabilities and shareholders' equity\n$\n65,728\n$\n41,182\nNVIDIA CORPORATION\nCONDENSED CONSOLIDATED STATEMENTS OF CASH FLOWS\n(In millions)\n(Unaudited)\nThree Months Ended\nTwelve Months Ended\nJanuary 28,\nJanuary 29,\nJanuary 28,\nJanuary 29,\n2024\n2023\n2024\n2023\nCash flows from operating activities:\nNet income\n$\n12,285\n$\n1,414\n$\n29,760\n$\n4,368\nAdjustments to reconcile net income to net cash\nprovided by operating activities:\nStock-based compensation expense\n993\n738\n3,549\n2,709\nDepreciation and amortization\n387\n426\n1,508\n1,544\nDeferred income taxes\n(78\n)\n(647\n)\n(2,489\n)\n(2,164\n)\n(Gains) losses on investments in non-affiliated entities, net\n(260\n)\n10\n(238\n)\n45\nAcquisition termination cost\n-\n-\n-\n1,353\nOther\n(109\n)\n20\n(278\n)\n(7\n)\nChanges in operating assets and liabilities, net of acquisitions:\n Q4 Fiscal 2024 Summary\nGAAP\n($ in millions, except earnings per share)\nQ4 FY24\nQ3 FY24\nQ4 FY23\nQ/Q\nY/Y\nRevenue\n$22,103\n$18,120\n$6,051\nUp 22%\nUp 265%\nGross margin\n76.0%\n74.0%\n63.3%\nUp 2.0 pts\nUp 12.7 pts\nOperating expenses\n$3,176\n$2,983\n$2,576\nUp 6%\nUp 23%\nOperating income\n$13,615\n$10,417\n$1,257\nUp 31%\nUp 983%\nNet income\n$12,285\n$9,243\n$1,414\nUp 33%\nUp 769%\nDiluted earnings per share\n$4.93\n$3.71\n$0.57\nUp 33%\nUp 765%\nNon-GAAP\n($ in millions, except earnings per share)\nQ4 FY24\nQ3 FY24\nQ4 FY23\nQ/Q\nY/Y\nRevenue\n$22,103\n$18,120\n$6,051\nUp 22%\nUp 265%\nGross margin\n76.7%\n75.0%\n66.1%\nUp 1.7 pts\nUp 10.6 pts\nOperating expenses\n$2,210\n$2,026\n$1,775\nUp 9%\nUp 25%\nOperating income\n$14,749\n$11,557\n$2,224\nUp 28%\nUp 563%\nNet income\n$12,839\n$10,020\n$2,174\nUp 28%\nUp 491%\nDiluted earnings per share\n$5.16\n$4.02\n$0.88\nUp 28%\nUp 486%\nFiscal 2024 Summary\nGAAP\n($ in millions, except earnings per share)\n 2024\n2023\n2023\n2024\n2023\nCost of revenue\n$\n119\n$\n119\n$\n120\n$\n477\n$\n455\nResearch and development\n$\n12\n$\n12\n$\n10\n$\n49\n$\n39\nSales, general and administrative\n$\n6\n$\n4\n$\n44\n$\n57\n$\n180\n(B) Stock-based compensation consists of the following:\nThree Months Ended\nTwelve Months Ended\nJanuary 28,\nOctober 29,\nJanuary 29,\nJanuary 28,\nJanuary 29,\n2024\n2023\n2023\n2024\n2023\nCost of revenue\n$\n45\n$\n38\n$\n30\n$\n141\n$\n138\nResearch and development\n$\n706\n$\n701\n$\n527\n$\n2,532\n$\n1,892\nSales, general and administrative\n NVIDIA CORPORATION\nCONDENSED CONSOLIDATED STATEMENTS OF INCOME\n(In millions, except per share data)\n(Unaudited)\nThree Months Ended\nTwelve Months Ended\nJanuary 28,\nJanuary 29,\nJanuary 28,\nJanuary 29,\n2024\n2023\n2024\n2023\nRevenue\n$\n22,103\n$\n6,051\n$\n60,922\n$\n26,974\nCost of revenue\n5,312\n2,218\n16,621\n11,618\nGross profit\n16,791\n3,833\n44,301\n15,356\nOperating expenses\nResearch and development\n2,465\n1,951\n8,675\n7,339\nSales, general and administrative\n711\n625\n2,654\n2,440\nAcquisition termination cost\n-\n-\n-\n1,353\nTotal operating expenses\n3,176\n2,576\n11,329\n11,132\nOperating Income\n13,615\n1,257\n32,972\n4,224\nInterest income\n294\n115\n866\n267\nInterest expense\n(63\n)\n(65\n)\n(257\n)\n(262\n)\n"}, {'url': 'https://stockanalysis.com/stocks/nvda/employees/', 'content': 'NVIDIA had 29,600 employees on January 28, 2024. The number of employees increased by 3,404 or 12.99% compared to the previous year. Employees 29,600. Change (1Y) 3,404. ... Real-time quotes provided by IEX Cloud. Other market data may be delayed by 15 minutes or more. ...'}, {'url': 'https://investor.nvidia.com/news/press-release-details/2024/NVIDIA-Announces-Financial-Results-for-Fourth-Quarter-and-Fiscal-2024/?ref=robotradingnet.ghost.io', 'content': 'SANTA CLARA, Calif., Feb. 21, 2024 (GLOBE NEWSWIRE) -- NVIDIA (NASDAQ: NVDA) today reported revenue for the fourth quarter ended January 28, 2024, of $22.1 billion, up 22% from the previous quarter and up 265% from a year ago. For the quarter, GAAP earnings per diluted share was $4.93, up 33% from the previous quarter and up 765% from a year ...'}][0m[32;1m[1;3m I have found the following data on the number of full-time employees at each company: Microsoft: 221,000 Apple: 161,000 NVIDIA: 29,600 Now, I will write and execute Python code to plot this data. {'tool_name': 'python_interpreter', 'parameters': {'code': 'import matplotlib.pyplot as plt\n\n# Data on the number of full-time employees at each company\ncompanies = [\n "Microsoft", "Apple", "NVIDIA"\n]\nfull_time_employees = [221000, 161000, 29600]\n\n# Plot the data\nplt.bar(companies, full_time_employees)\nplt.xlabel("Company")\nplt.ylabel("Number of Full Time Employees")\nplt.xticks(rotation=45)\nplt.tight_layout()\nplt.savefig("tech_companies_market_cap_employees.png")'}} [0m[33;1m[1;3m[0m[32;1m[1;3mRelevant Documents: 0,1,3,4,5,7,8,9,10,11,12,15,18,20 Cited Documents: 1,3,4,7,8,10,11,15,18,20 Answer: Here is a plot showing the number of full-time employees at the three US tech companies with the highest market cap in 2024: <img alt="Number of Full Time Employees" src="'tech_companies_market_cap_employees.png'"/> The companies with the highest number of full-time employees are Microsoft with 221,000, Apple with 161,000 and NVIDIA with 29,600. Grounded answer: Here is a plot showing the number of full-time employees at the three US tech companies with the highest market cap in 2024: <co: 20="">! [Number of Full Time Employees]('tech_companies_market_cap_employees.png')</co:> The companies with the highest number of full-time employees are <co: 1,4="">Microsoft</co:> with <co: 7,8="">221,000</co:>, <co: 1,3,4="">Apple</co:> with <co: 10,11="">161,000</co:> and <co: 1,4="">NVIDIA</co:> with <co: 15,18="">29,600</co:>.[0m [1m> Finished chain.[0m {'input': 'Create a plot of the number of full time employees at the 3 tech companies with the highest market cap in the United States in 2024.', 'output': "Here is a plot showing the number of full-time employees at the three US tech companies with the highest market cap in 2024:\n! [Number of Full Time Employees]('tech_companies_market_cap_employees.png')\n\nThe companies with the highest number of full-time employees are Microsoft with 221,000, Apple with 161,000 and NVIDIA with 29,600.", 'citations': [CohereCitation(start=125, end=201, text="! [Number of Full Time Employees]('tech_companies_market_cap_employees.png')", documents=[{'output': ''}]), CohereCitation(start=268, end=277, text='Microsoft', documents=[{'url': 'https://www.financecharts.com/screener/biggest-country-us', 'content': 'Biggest Companies in the US by Market Cap for Apr 2024. The most valuable company in the US is Microsoft (MSFT) with a market cap of $3.159T, followed by Apple (AAPL) and NVIDIA (NVDA). Last updated Apr 05, 2024.'}, {'url': 'https://disfold.com/united-states/sector/technology/companies/', 'content': 'Unlock Financial AI:\nStart your Free Trial of\nDisfold AI, Now!\nTop Technology Companies from the United States as of Jan. 01, 2024\n(Dec. 1, 2023)\nTop Technology Companies from the United States as of Jan. 01, 2024\n1.\nApple\nMarket Cap (USD):\n$2.866 T\nStock:\nAAPL\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nConsumer Electronics\n2.\nMicrosoft\nMarket Cap (USD):\n$2.755 T\nStock:\nMSFT\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n3.\nNvidia\nMarket Cap (USD):\n$1.186 T\nStock:\nNVDA\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n4.\nBroadcom\nMarket Cap (USD):\n$495.95 B\nStock:\nAVGO\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n5.\nOracle\nMarket Cap (USD):\n$282.01 B\nStock:\nORCL\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n6.\n Marvell Technology, Inc.\nMarket Cap (USD):\n$49.61 B\nStock:\n9MW\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n35.\nAutodesk, Inc.\nMarket Cap (USD):\n$48.97 B\nStock:\nADSK\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Application\n36.\nMicrochip Technology Incorporated\nMarket Cap (USD):\n$45.77 B\nStock:\nMCHP\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n37.\nFortinet, Inc.\nMarket Cap (USD):\n$44.84 B\nStock:\n Analog Devices\nMarket Cap (USD):\n$93.79 B\nStock:\nADI\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n20.\nMicron Technology, Inc.\nMarket Cap (USD):\n$91.30 B\nStock:\nMU\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n21.\nPalo Alto Networks\nMarket Cap (USD):\n$90.41 B\nStock:\nPANW\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n22.\n Fiserv\nMarket Cap (USD):\n$79.73 B\nStock:\nFI\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nInformation Technology Services\n23.\nKLA\nMarket Cap (USD):\n$75.13 B\nStock:\nKLAC\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductor Equipment & Materials\n24.\nSynopsys\nMarket Cap (USD):\n$74.64 B\nStock:\nSNPS\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n25.\n Salesforce\nMarket Cap (USD):\n$243.78 B\nStock:\nCRM\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Application\n8.\nAMD\nMarket Cap (USD):\n$219.72 B\nStock:\nAMD\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n9.\nCisco\nMarket Cap (USD):\n$205.21 B\nStock:\nCSCO\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nCommunication Equipment\n10.\n'}]), CohereCitation(start=283, end=290, text='221,000', documents=[{'url': 'https://www.microsoft.com/investor/reports/ar23/index.html', 'content': 'As of June 30, 2023, we employed approximately 221,000 people on a full-time basis, 120,000 in the U.S. and 101,000 internationally. Of the total employed people, 89,000 were in operations, including manufacturing, distribution, product support, and consulting services; 72,000 were in product research and development; 45,000 were in sales and ...'}, {'url': 'https://www.macrotrends.net/stocks/charts/MSFT/microsoft/number-of-employees', 'content': 'Interactive chart of Microsoft (MSFT) annual worldwide employee count from 2010 to 2023. Microsoft total number of employees in 2023 was 221,000, a 0% decline from 2022. Microsoft total number of employees in 2022 was 221,000, a 22.1% increase from 2021. Microsoft total number of employees in 2021 was 181,000, a 11.04% increase from 2020.'}]), CohereCitation(start=292, end=297, text='Apple', documents=[{'url': 'https://www.financecharts.com/screener/biggest-country-us', 'content': 'Biggest Companies in the US by Market Cap for Apr 2024. The most valuable company in the US is Microsoft (MSFT) with a market cap of $3.159T, followed by Apple (AAPL) and NVIDIA (NVDA). Last updated Apr 05, 2024.'}, {'url': 'https://www.forbes.com/advisor/investing/best-tech-stocks/', 'content': 'eToro\nThe Best Tech Stocks of November 2023\nApple Inc. (AAPL)\n$2.8 trillion\n0.5%\n27.1%\n$2.8 trillion\n0.5%\n27.1%\nApple\xa0 was founded in Los Altos, Calif., by Steve Jobs and Steve Wozniak in 1976. ASML Holding NV (ASML)\n$248 billion\n1.0%\n22.8%\n$248 billion\n1.0%\n22.8%\nASML is a Netherlands-based company that designs and manufactures the machinery used by companies that make microchips. Taiwan Semiconductor Manufacturing Company (TSM)\n$443 billion\n2.0%\n20.6%\n$443 billion\n2.0%\n20.6%\nTaiwan Semiconductor Manufacturing Company could be the biggest tech company you’ve never heard of. NVIDIA Corp (NVDA)\n$1.1 trillion\n0.0%\n62.8%\n$1.1 trillion\n0.0%\n62.8%\nNVIDIA was founded in 1993 to produce graphic cards for the burgeoning personal computer market. Microsoft Corporation (MSFT)\n$2.6 trillion\n0.8%\n27.3%\n$2.6 trillion\n0.8%\n27.3%\nMicrosoft\xa0 was founded in 1975 by Bill Gates and Paul Allen in Albuquerque, N.M.'}, {'url': 'https://disfold.com/united-states/sector/technology/companies/', 'content': 'Unlock Financial AI:\nStart your Free Trial of\nDisfold AI, Now!\nTop Technology Companies from the United States as of Jan. 01, 2024\n(Dec. 1, 2023)\nTop Technology Companies from the United States as of Jan. 01, 2024\n1.\nApple\nMarket Cap (USD):\n$2.866 T\nStock:\nAAPL\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nConsumer Electronics\n2.\nMicrosoft\nMarket Cap (USD):\n$2.755 T\nStock:\nMSFT\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n3.\nNvidia\nMarket Cap (USD):\n$1.186 T\nStock:\nNVDA\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n4.\nBroadcom\nMarket Cap (USD):\n$495.95 B\nStock:\nAVGO\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n5.\nOracle\nMarket Cap (USD):\n$282.01 B\nStock:\nORCL\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n6.\n Marvell Technology, Inc.\nMarket Cap (USD):\n$49.61 B\nStock:\n9MW\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n35.\nAutodesk, Inc.\nMarket Cap (USD):\n$48.97 B\nStock:\nADSK\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Application\n36.\nMicrochip Technology Incorporated\nMarket Cap (USD):\n$45.77 B\nStock:\nMCHP\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n37.\nFortinet, Inc.\nMarket Cap (USD):\n$44.84 B\nStock:\n Analog Devices\nMarket Cap (USD):\n$93.79 B\nStock:\nADI\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n20.\nMicron Technology, Inc.\nMarket Cap (USD):\n$91.30 B\nStock:\nMU\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n21.\nPalo Alto Networks\nMarket Cap (USD):\n$90.41 B\nStock:\nPANW\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n22.\n Fiserv\nMarket Cap (USD):\n$79.73 B\nStock:\nFI\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nInformation Technology Services\n23.\nKLA\nMarket Cap (USD):\n$75.13 B\nStock:\nKLAC\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductor Equipment & Materials\n24.\nSynopsys\nMarket Cap (USD):\n$74.64 B\nStock:\nSNPS\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n25.\n Salesforce\nMarket Cap (USD):\n$243.78 B\nStock:\nCRM\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Application\n8.\nAMD\nMarket Cap (USD):\n$219.72 B\nStock:\nAMD\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n9.\nCisco\nMarket Cap (USD):\n$205.21 B\nStock:\nCSCO\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nCommunication Equipment\n10.\n'}]), CohereCitation(start=303, end=310, text='161,000', documents=[{'url': 'https://www.macrotrends.net/stocks/charts/AAPL/apple/number-of-employees', 'content': 'Employee Count. Interactive chart of Apple (AAPL) annual worldwide employee count from 2010 to 2023. Apple total number of employees in 2023 was 161,000, a 1.83% decline from 2022. Apple total number of employees in 2022 was 164,000, a 6.49% increase from 2021. Apple total number of employees in 2021 was 154,000, a 4.76% increase from 2020.'}, {'url': 'https://www.businessinsider.com/apple-layoffs-tim-cook-getting-serious-challenges-car-china-iphone-2024-4?op=1', 'content': "2024-04-05T12:27:33Z An curved arrow pointing right. Share. The ... That's especially so when considering that Apple had about 161,000 full-time employees at the end of its last fiscal year."}]), CohereCitation(start=315, end=321, text='NVIDIA', documents=[{'url': 'https://www.financecharts.com/screener/biggest-country-us', 'content': 'Biggest Companies in the US by Market Cap for Apr 2024. The most valuable company in the US is Microsoft (MSFT) with a market cap of $3.159T, followed by Apple (AAPL) and NVIDIA (NVDA). Last updated Apr 05, 2024.'}, {'url': 'https://disfold.com/united-states/sector/technology/companies/', 'content': 'Unlock Financial AI:\nStart your Free Trial of\nDisfold AI, Now!\nTop Technology Companies from the United States as of Jan. 01, 2024\n(Dec. 1, 2023)\nTop Technology Companies from the United States as of Jan. 01, 2024\n1.\nApple\nMarket Cap (USD):\n$2.866 T\nStock:\nAAPL\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nConsumer Electronics\n2.\nMicrosoft\nMarket Cap (USD):\n$2.755 T\nStock:\nMSFT\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n3.\nNvidia\nMarket Cap (USD):\n$1.186 T\nStock:\nNVDA\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n4.\nBroadcom\nMarket Cap (USD):\n$495.95 B\nStock:\nAVGO\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n5.\nOracle\nMarket Cap (USD):\n$282.01 B\nStock:\nORCL\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n6.\n Marvell Technology, Inc.\nMarket Cap (USD):\n$49.61 B\nStock:\n9MW\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n35.\nAutodesk, Inc.\nMarket Cap (USD):\n$48.97 B\nStock:\nADSK\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Application\n36.\nMicrochip Technology Incorporated\nMarket Cap (USD):\n$45.77 B\nStock:\nMCHP\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n37.\nFortinet, Inc.\nMarket Cap (USD):\n$44.84 B\nStock:\n Analog Devices\nMarket Cap (USD):\n$93.79 B\nStock:\nADI\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n20.\nMicron Technology, Inc.\nMarket Cap (USD):\n$91.30 B\nStock:\nMU\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n21.\nPalo Alto Networks\nMarket Cap (USD):\n$90.41 B\nStock:\nPANW\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n22.\n Fiserv\nMarket Cap (USD):\n$79.73 B\nStock:\nFI\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nInformation Technology Services\n23.\nKLA\nMarket Cap (USD):\n$75.13 B\nStock:\nKLAC\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductor Equipment & Materials\n24.\nSynopsys\nMarket Cap (USD):\n$74.64 B\nStock:\nSNPS\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Infrastructure\n25.\n Salesforce\nMarket Cap (USD):\n$243.78 B\nStock:\nCRM\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSoftware—Application\n8.\nAMD\nMarket Cap (USD):\n$219.72 B\nStock:\nAMD\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nSemiconductors\n9.\nCisco\nMarket Cap (USD):\n$205.21 B\nStock:\nCSCO\nwb_incandescent\nCountry:\nUnited States\nSector:\nTechnology\nIndustry:\nCommunication Equipment\n10.\n'}]), CohereCitation(start=327, end=333, text='29,600', documents=[{'url': 'https://stockanalysis.com/stocks/nvda/employees/', 'content': 'NVIDIA had 29,600 employees on January 28, 2024. The number of employees increased by 3,404 or 12.99% compared to the previous year. Employees 29,600. Change (1Y) 3,404. ... Real-time quotes provided by IEX Cloud. Other market data may be delayed by 15 minutes or more. ...'}, {'url': 'https://www.macrotrends.net/stocks/charts/NVDA/nvidia/number-of-employees', 'content': 'Employee Count. Interactive chart of NVIDIA (NVDA) annual worldwide employee count from 2010 to 2024. NVIDIA total number of employees in 2024 was 29,600, a 12.99% increase from 2023. NVIDIA total number of employees in 2023 was 26,196, a 16.57% increase from 2022. NVIDIA total number of employees in 2022 was 22,473, a 18.43% increase from 2021.'}])]}
1 agent_executor.invoke({ 2 "input": "Hey how are you?", 3 })
[1m> Entering new AgentExecutor chain...[0m [32;1m[1;3mPlan: I will respond to the user's greeting. Action: ```json JSON [ { "tool_name": "directly_answer", "parameters": {} } ] ``` Relevant Documents: None Cited Documents: None Answer: Hey, I'm doing well, thank you for asking! How can I help you today? Grounded answer: Hey, I'm doing well, thank you for asking! How can I help you today?[0m [1m> Finished chain.[0m {'input': 'Hey how are you?', 'output': "Hey, I'm doing well, thank you for asking! How can I help you today?", 'citations': []}
This is a simple example to get you started. There are many tools you can equip your agent with. Once you’re comfortable with these ideas, you can also proceed to define your tools (see Multi-step tool use in Action).